Using vuejs-datepicker with Nuxt JS
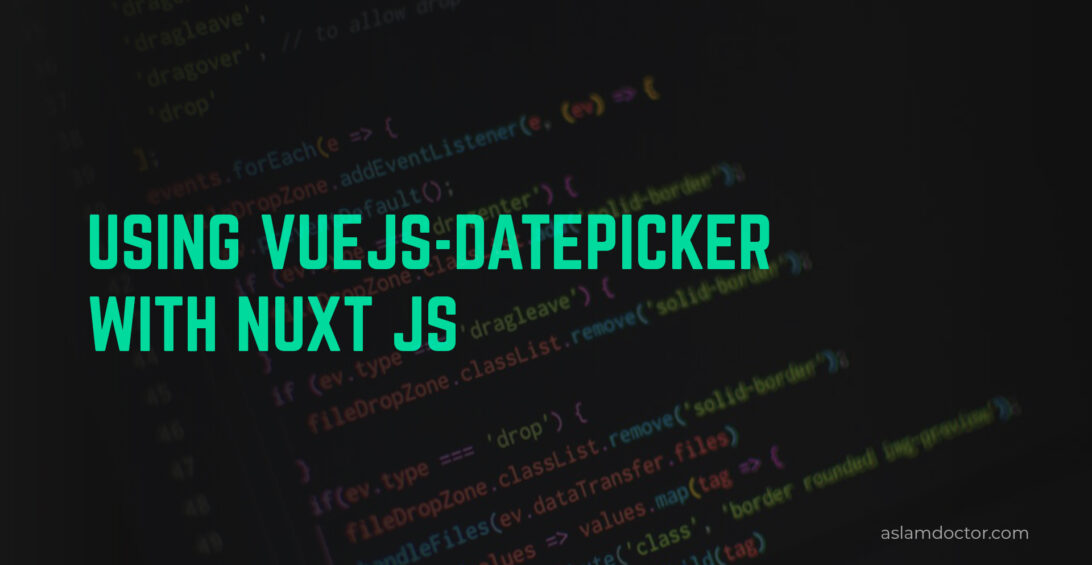
Talking about date pickers, if we check on npmjs.com, the most downloaded datepicker component for Vue JS is vuejs-datepicker. No doubt, the plugin(component) has every essential feature any datepicker should have.
But when it comes to integrating it with Nuxt JS framework, the plugin breaks the whole page because it doesn’t support SSR (server-side rendering via Node JS). In fact, most of the datepicker plugins don’t support SSR. After some reading in the documentation and tweaking the code, I found a way for this to work perfectly even without SSR Support.
Nuxt JS comes with a feature inbuilt that allows loading a component on client-side only. To do that, all you need to do is simply place your component inside <client-only> tags. And it should work. Below are the steps I followed to integrate vuejs-datepicker with Nuxt JS.
1. Install the vuejs-datepicker
npm i vuejs-datepicker
2. Create a plugin file inside your nuxt project under /plugins/vue-datepicker.js And add the below code to it.
import Vue from 'vue' import Datepicker from 'vuejs-datepicker' Vue.component('date-picker', Datepicker)
And add a line under nuxt.config.js file inside the plugins section like this :
plugins: [ ... other plugins { src: '~/plugins/vue-datepicker', ssr: false }, // datepicker plugin here ],
This way we are globally registering the component named <date-picker>.
3. Now to add components to your pages or any other components, just add a code like this wherever you want the datepicker to be loaded.
<client-only><date-picker placeholder="MM/DD/YYYY" format="MM/dd/yyyy" v-model="date_today" /></client-only>
And inside your Vue data(), define a date_today variable as below
data(){ return{ ... your other data variables ... date_today:new Date() } }
If you are using a version of Nuxt < v2.9.0, use <no-ssr> instead of <client-only>, And this will perfectly load the datepicker component with the current date as the default value. Hope you guys found this article helpful. Please share it with other developers.
Ref: https://nuxtjs.org/api/components-client-only#the-lt-client-only-gt-component