Thursday, December 22, 2022
My WooCommerce Cheatsheet
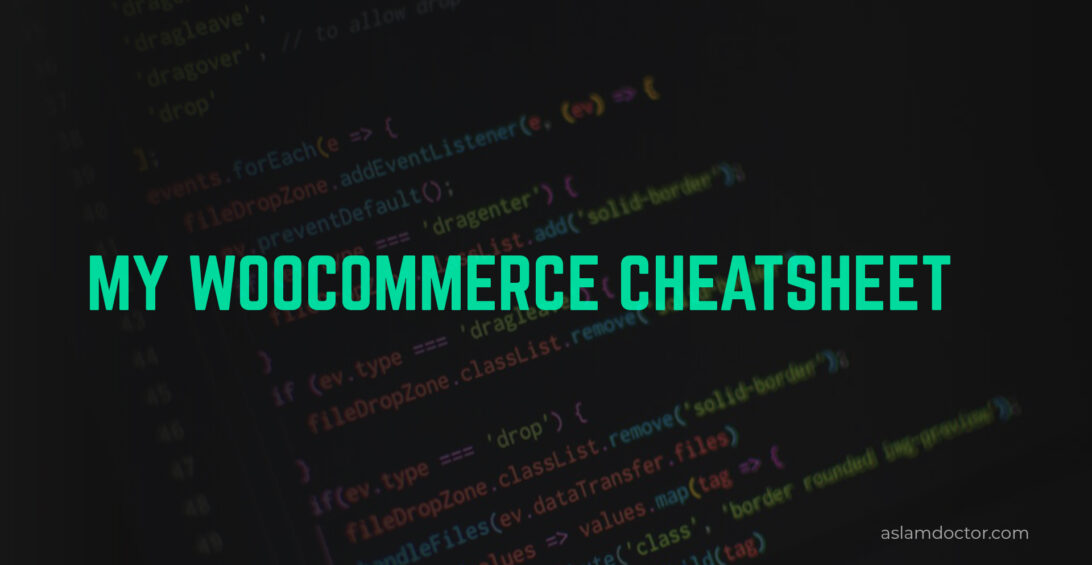
These are some of the common codes that I use in any WordPress WooCommerce-based project. Hope you guys find it useful.
Add WooCommerce template customization support in the theme
add_theme_support( 'woocommerce', array( // 'thumbnail_image_width' => 200, // 'gallery_thumbnail_image_width' => 100, // 'single_image_width' => 470, ) );
Get The WooCommerce Page ID You Need
get_option( 'woocommerce_shop_page_id' ); get_option( 'woocommerce_cart_page_id' ); get_option( 'woocommerce_checkout_page_id' ); get_option( 'woocommerce_pay_page_id' ); get_option( 'woocommerce_thanks_page_id' ); get_option( 'woocommerce_myaccount_page_id' ); get_option( 'woocommerce_edit_address_page_id' ); get_option( 'woocommerce_view_order_page_id' ); get_option( 'woocommerce_terms_page_id' );
Enable product gallery, zoom, lightbox
add_theme_support( 'wc-product-gallery-slider' ); add_theme_support( 'wc-product-gallery-zoom' ); add_theme_support( 'wc-product-gallery-lightbox' );
Add specific CSS class to the body tag for NON-WooCommerce Pages
add_filter( 'body_class', 'wpmix_body_class' ); function wpmix_body_class( $classes ) { if ( ! is_woocommerce() ) { $classes[] = 'woocommerce notWoo'; } return $classes; }
Remove WooCommerce Sidebar
remove_action( 'woocommerce_sidebar', 'woocommerce_get_sidebar', 10 );
Remove Related Products Section
remove_action( 'woocommerce_after_single_product_summary', 'woocommerce_output_related_products', 20 );
Remove Category names from Single Product Summary
remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_meta', 40 );
Remove Upsells
remove_action( 'woocommerce_after_single_product_summary', 'woocommerce_upsell_display', 15 );
Add Title as Alt tags on all product images
add_filter( 'wp_get_attachment_image_attributes', 'wpmix_change_attachement_image_attributes', 20, 2 ); function wpmix_change_attachement_image_attributes( $attr, $attachment ) { // Get post parent. $parent = get_post_field( 'post_parent', $attachment ); // Get post type to check if it's product. $type = get_post_field( 'post_type', $parent ); if ( $type != 'product' ) { return $attr; } // Get title $title = get_post_field( 'post_title', $parent ); $attr['alt'] = $title; $attr['title'] = $title; return $attr; }
Update cart counter when product added to cart
add_filter( 'woocommerce_add_to_cart_fragments', 'wpmix_cart_count_fragments', 10, 1 ); function wpmix_cart_count_fragments( $fragments ) { $fragments['a.cart-link span.count'] = '<span class="count">' . WC()->cart->get_cart_contents_count() . '</span>'; return $fragments; }
Custom checkout button
add_action( 'woocommerce_widget_shopping_cart_buttons', 'wpmix_proceed_to_checkout', 22 ); function wpmix_proceed_to_checkout() { echo '<a href="' . wc_get_checkout_url() . '" class="btn btn-black btn-block text-uppercase">Proceed to Checkout</a>'; }
Remove the company name field from the checkout page
add_filter( 'woocommerce_checkout_fields', 'wpmix_override_checkout_fields' ); function wpmix_override_checkout_fields( $fields ) { unset( $fields['shipping']['billing_company'] ); return $fields; }
Remove different links from My Account
add_filter( 'woocommerce_account_menu_items', 'wpmix_remove_my_account_links' ); function wpmix_remove_my_account_links( $menu_links ) { unset( $menu_links['subscriptions'] ); // Subscriptions unset( $menu_links['payment-methods'] ); // Payment methods unset( $menu_links['downloads'] ); // Payment methods return $menu_links; }
Move the price below the Short description on the Product Page
remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_price', 4 ); remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_price', 10 ); add_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_price', 29 );
Remove tags from the Product Page
add_action( 'after_setup_theme', 'wpmix_after_setup_theme' ); function wpmix_after_setup_theme() { remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_meta', 40 ); }
Remove the breadcrumb
add_action( 'init', 'wpmix_remove_wc_breadcrumbs' ); function wpmix_remove_wc_breadcrumbs() { remove_action( 'woocommerce_before_main_content', 'woocommerce_breadcrumb', 20, 0 ); }
Remove the product details tab
add_filter( 'woocommerce_product_tabs', 'wpmix_remove_description_tab', 11 ); function wpmix_remove_description_tab( $tabs ) { unset( $tabs['additional_information'] ); return $tabs; }
Rename product tab titles
add_filter( 'woocommerce_product_tabs', 'wpmix_rename_additional_info_tab' ); function wpmix_rename_additional_info_tab( $tabs ) { $tabs['description']['title'] = 'Description'; $tabs['short_description']['title'] = 'More Information'; return $tabs; }
Modify product sorting options in the dropdown
add_filter( 'woocommerce_catalog_orderby', 'wpmix_customize_product_sorting' ); function wpmix_customize_product_sorting( $sorting_options ) { $sorting_options = array( 'menu_order' => __( 'Sort', 'woocommerce' ), 'popularity' => __( 'Popularity', 'woocommerce' ), 'rating' => __( 'Average rating', 'woocommerce' ), 'date' => __( 'Newness', 'woocommerce' ), 'price' => __( 'Price: low to high', 'woocommerce' ), 'price-desc' => __( 'Price: high to low', 'woocommerce' ), ); return $sorting_options; }
Order products by Stock status (show out of stock last)
add_filter( 'woocommerce_get_catalog_ordering_args', 'wpmix_sort_by_stock_amount', 9999 ); function wpmix_sort_by_stock_amount( $args ) { $args['orderby'] = array( 'meta_value' => 'ASC', 'menu_order' => 'ASC', ); $args['meta_key'] = '_stock_status'; return $args; }
Limit orders by a specific amount
add_action( 'woocommerce_check_cart_items', 'wpmix_set_min_total' ); function wpmix_set_min_total() { // Only run in the Cart or Checkout pages if ( is_cart() || is_checkout() ) { global $woocommerce; // Set minimum cart total $maximum_cart_total = 150; // A Maximum of 150 amount is required before checking out. $total = WC()->cart->subtotal; // Compare values and add an error is Cart's total if ( $total > $maximum_cart_total ) { // Display our error message wc_add_notice( sprintf( 'Total cost of products in cart must be <strong>£150.00</strong> or less.' . '<br /><br />Current cart\'s total: <strong>£%s</strong>', $total ), 'error' ); } } }